IT Professional Curricula Software Development Solution Area Generic Languages C++ Programming
The C++ Standard Template Library is a set of template classes that let you easily implement common algorithms and data structures. Explore the Standard Library algorithms and operations, including sorting and merging.
Objectives |
---|
Nonmodifying Sequence Operations in C++
Modifying Sequence Operations in C++
STL Sorting Operations
STL Merging Algorithms
Practice: Algorithms and Operations
|
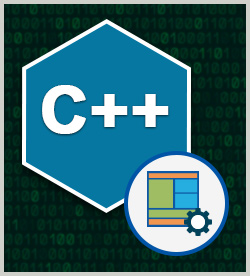