IT Professional Curricula Software Development Solution Area Microsoft F# F# Fundamentals
F# is a Microsoft .NET language that offers support for functional programming in addition to object-oriented and imperative programming. This course introduce F#, describes the templates for creating F# programs and files in Visual Studio, and describes how to implement functional and object-oriented programming with F#. You'll also learn how to use conditional expressions, work with loops, and handle exceptions with F#.
Objectives |
---|
Introducing F# and Programming Functions
Working with Functions
Functional Types and Mutability
Program Flow Control
Object Oriented Programming in F#
Interfaces and Inheritance
Static and Abstract Classes
Working with Methods
Practice: Functions and Objects in F# Programs
|
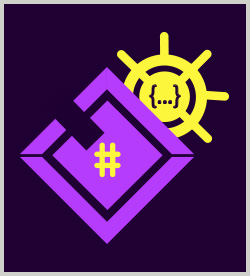